Lab 6: Road Detection with Threads (2 pts)
Chris Tralie
Due Wednesday 10/28/2020
Overview / Logistics
The purpose of this lab is to give you practice with the basics of threads in Java. Click here to download the Netbeans starter code for this lab. When you are finished, please upload your modified Hough.java
to canvas, as well as a README.txt file with timings running the code with only one thread on your computer versus with multiple threads. If you've done this properly, you should see a speedup when running it with multiple threads, as virtually all computers these days have multiple cores.
Learning Objectives
- Make slight tweaks to a fully functioning codebase that someone else wrote to make it run faster.
- Practice using threads and synchronization in Java
Background / Programming Task
Autonomous vehicle engineering has been in progress for decades, and has recently seen major improvements with the advent of more robust computer vision systems. In this lab, you will examine a very simple (not necessarily state of the art) part of such a system that works to detect roads. You have been provided with a naive implementation of code that detects lines in an image, which include the road boundaries and lane markers. This code first creates a "gradient image" (high intensity for large changes around edges), followed by a "thinned edge image" (See my writeup about this process back from 2009, back when I was an undergraduate taking computer vision). Then, it checks all possible lines and sums up how much energy in the thinned edge image is in each one, and it keeps the lines above a certain threshold. The animation below shows this process
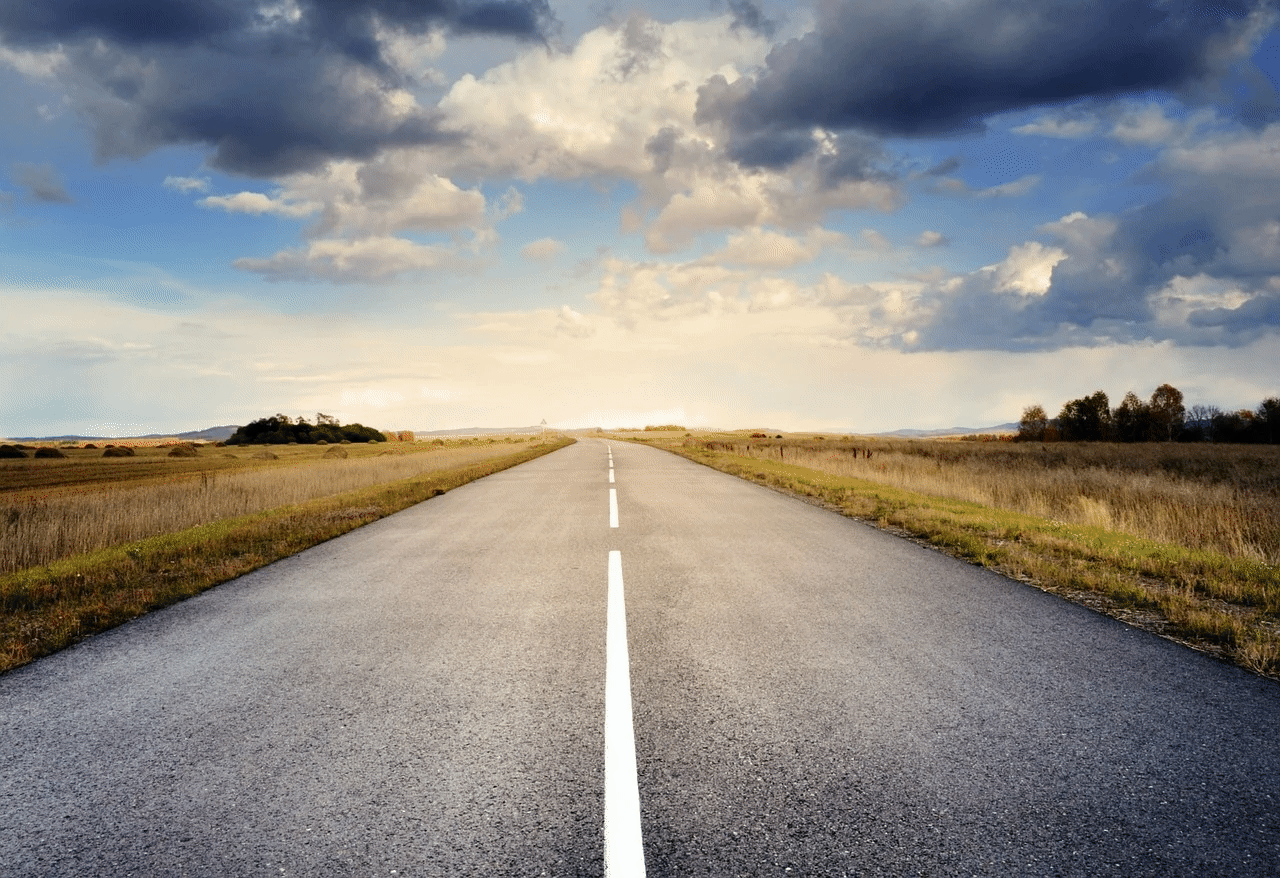
Unfortunately, as written, this process is slow, since it's a triply nested loop, as seen in the getEdges()
method of Hough.java
:
- For each angle the line can be
-
For each shift of the line from one corner of the image to the opposite corner
- Sum up each pixel on the line
-
For each shift of the line from one corner of the image to the opposite corner
But the process to sum up the energy on each line can be parallelized easily, since the lines don't depend on each other. Therefore, your job will be to use threads to make this run faster, and you should report evidence in a README file of timings to show that this is indeed the case. The easiest way to modify your code to run with threads in parallel is to split up the angles that the program checks and put different ranges of angles on different threads. Here are some hints:
-
The main method in
Hough.java
takes two command line arguments. The first is a path to the image on which lines are being detected, and the second is the minimum energy threshold at which to keep a line. For example will yield the image shown above. Larger thresholds will lead to fewer lines. -
You will need to make the
Hough
class implement theRunnable
interface, and you will need to make the heavy lifting occur in thepublic void run()
method. You will then need to setup differentHough
objects to run in separate threads. Refer to our examples from class for help with this. -
Thread collisions can occur when they go to draw lines whose energy is above a particular threshold, so you should
synchronize
the image when you go to do the draw. Refer again to our class example on this.